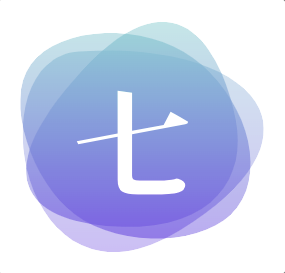
Java8函数式接口-Predicate以及Operator
Java8函数式接口-Predicate以及Operator
Predicate
和 Operator
也是比较常用的函数式接口。 在以往的项目实践中,Predicate
主要用于过滤和匹配。Operator
接口用于对参数进行转换或者合并等。
1. Operator Interfaces 操作符接口
这些接口的行为类似于 Java 中的一元运算符或二元运算符,常用于做数据加减乘除、累加等等。
1.1 UnaryOperator
Unary 在英语中指的是【单一的】或【一个的】的意思,组合下意思就是单一参数的操作。
源码:
@FunctionalInterface
public interface UnaryOperator<T> extends Function<T, T> {
static <T> UnaryOperator<T> identity() {
return t -> t;
}
}
作用: 接收 一个 参数并产生一个相同类型结果的操作。它继承自 Function
运用场景: 对一个参数进行转换或修改 , 并且转换后的参数类型与原参数类型相同, 就可以可以使用 UnaryOperator
final UnaryOperator<String> toUpperCase = String::toUpperCase;
// 结果为 "HELLO"
final String result = toUpperCase.apply("hello");
1.2 BinaryOperator
binary 在英语里的意思是【双的】或者【两个的】,组合起来就是两个参数的操作。
源码:
@FunctionalInterface
public interface BinaryOperator<T> extends BiFunction<T,T,T> {
public static <T> BinaryOperator<T> minBy(Comparator<? super T> comparator) {
Objects.requireNonNull(comparator);
return (a, b) -> comparator.compare(a, b) <= 0 ? a : b;
}
public static <T> BinaryOperator<T> maxBy(Comparator<? super T> comparator) {
Objects.requireNonNull(comparator);
return (a, b) -> comparator.compare(a, b) >= 0 ? a : b;
}
}
作用: 接收 两个 相同类型的参数并产生一个相同类型结果的操作。继承 BiFunction
运用场景: 对两个相同类型的对象进行加减乘除、比较或者计算的场景下 , 返回结果类型也与输入对象类型相同的时候 , 可以用 BinaryOperator
final BinaryOperator<Integer> sumOperator = Integer::sum;
// 结果为 12
final int sum = sumOperator.apply(5, 7);
注意上面两个接口的差异在于一个参数跟两个参数,通过接口名前面的单词进行区分。
2. Predicate Interfaces 谓词接口
这些接口通常用于对对象进行过滤,或者测试条件 , 检查其是否符合特定的条件或属性,然后返回一个布尔值。
比如在 Java 8 的 Stream API 中 filter()
方法就可以接收一个 Predicate
2.1 Predicate
源码:
@FunctionalInterface
public interface Predicate<T> {
boolean test(T t);
default Predicate<T> and(Predicate<? super T> other) {
Objects.requireNonNull(other);
return (t) -> test(t) && other.test(t);
}
default Predicate<T> negate() {
return (t) -> !test(t);
}
default Predicate<T> or(Predicate<? super T> other) {
Objects.requireNonNull(other);
return (t) -> test(t) || other.test(t);
}
static <T> Predicate<T> isEqual(Object targetRef) {
return (null == targetRef)
? Objects::isNull
: object -> targetRef.equals(object);
}
}
作用: 接收一个参数,执行判断条件后返回一个布尔值。最常用的就是 test()
方法。
使用场景: 需要对参数值或者参数对象进行某种条件判断或过滤时。
// 判断传入的参数是不是偶数
final Predicate<Integer> isEven = x -> x % 2 == 0;
// 结果为 true
final boolean result = isEven.test(4);
2.2 BiPredicate
源码:
@FunctionalInterface
public interface BiPredicate<T, U> {
boolean test(T t, U u);
default BiPredicate<T, U> and(BiPredicate<? super T, ? super U> other) {
Objects.requireNonNull(other);
return (T t, U u) -> test(t, u) && other.test(t, u);
}
default BiPredicate<T, U> negate() {
return (T t, U u) -> !test(t, u);
}
default BiPredicate<T, U> or(BiPredicate<? super T, ? super U> other) {
Objects.requireNonNull(other);
return (T t, U u) -> test(t, u) || other.test(t, u);
}
}
作用: 接收 两个参数 并返回一个布尔值的操作。
使用场景:需要对 两个不同类型的参数 进行某种条件测试或过滤的时候 。
// 判断传进来的字符串长度是不是超过目标长度值
final BiPredicate<String, Integer> lengthGreaterThan = (str, len) -> str.length() > len;
// 传入的字符串长度为5,目标长度值为3 结果为 true
boolean result = lengthGreaterThan.test("Hello", 3);
2.3 DoublePredicate
源码:
@FunctionalInterface
public interface DoublePredicate {
boolean test(double value);
default DoublePredicate and(DoublePredicate other) {
Objects.requireNonNull(other);
return (value) -> test(value) && other.test(value);
}
default DoublePredicate negate() {
return (value) -> !test(value);
}
default DoublePredicate or(DoublePredicate other) {
Objects.requireNonNull(other);
return (value) -> test(value) || other.test(value);
}
}
作用: 接收一个 double 类型的参数,然后返回一个布尔值的操作。
使用场景: 对 double 类型的值进行某种条件测试或过滤时。
// 测试是不是正数
final DoublePredicate isPositive = x -> x > 0;
// 结果为 true
boolean result = isPositive.test(3.14);
2.4 IntPredicate
源码:
@FunctionalInterface
public interface IntPredicate {
boolean test(int value);
default IntPredicate and(IntPredicate other) {
Objects.requireNonNull(other);
return (value) -> test(value) && other.test(value);
}
default IntPredicate negate() {
return (value) -> !test(value);
}
default IntPredicate or(IntPredicate other) {
Objects.requireNonNull(other);
return (value) -> test(value) || other.test(value);
}
}
作用:接收一个 int 类型的参数,然后返回一个布尔值的操作。
使用场景:对 int 类型的值进行某种条件测试或过滤时。
// 判断 Int 数值是不是偶数
final IntPredicate isEven = x -> x % 2 == 0;
final boolean result = isEven.test(4);
2.5 LongPredicate
源码:
@FunctionalInterface
public interface LongPredicate {
boolean test(long value);
default LongPredicate and(LongPredicate other) {
Objects.requireNonNull(other);
return (value) -> test(value) && other.test(value);
}
default LongPredicate negate() {
return (value) -> !test(value);
}
default LongPredicate or(LongPredicate other) {
Objects.requireNonNull(other);
return (value) -> test(value) || other.test(value);
}
}
作用:接收一个 long 类型的参数,然后返回一个布尔值的操作。
使用场景:对 long 类型的值进行某种条件测试或过滤时。
final LongPredicate isPositive = x -> x > 0;
final boolean result = isPositive.test(123L);