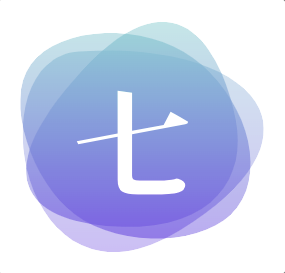
Java8函数式接口-Consumer
Java8函数式接口-Consumer
这些接口通常用于对输入参数执行某些无返回值的操作 , 例如打印日志、调试输出、保存数据等一些业务C操作。
跟 Function 接口的差异点在于,Consumer 接口只接收参数不返回结果,Function 是又接收参数又有返回值。
Consumer 在 Java 8 的 Stream API 中用的也比较多 , 比如 forEach()
方法就可以接收一个 Consumer
1. 通用的 Consumer 接口
JDK 提供的通用的 Consumer 接口目前为止一共有下面 8 个。
Consumer<T>
BiConsumer<T, U>
IntConsumer
LongConsumer
DoubleConsumer
ObjIntConsumer<T>
ObjLongConsumer<T>
ObjDoubleConsumer<T>
跟其他接口的套路还是类似。 根据命名可以很容易可以猜的出来套路,这些接口都是没有返回值的。
- Cosumer 是接收一个入参然后执行操作。
- BiConsumer 是接收两个入参然后执行操作。
- IntConsumer、LongConsumer、DoubleConsumer 是分别接收对应的类型参数然后执行操作。
- ObjDoubleConsumer、ObjIntConsumer、ObjLongConsumer 是接收一个 Object 类型的参数以及一个对应类型的参数,然后执行操作。
2. 通用 Consumer 接口定义
4.4.1 Consumer
源码:
@FunctionalInterface
public interface Consumer<T> {
/**
* Performs this operation on the given argument.
*
* @param t the input argument
*/
void accept(T t);
default Consumer<T> andThen(Consumer<? super T> after) {
Objects.requireNonNull(after);
return (T t) -> { accept(t); after.accept(t); };
}
}
使用场景: 接收 一个任意类型的参数 并且不返回任何结果的操作。通常是对传入的参数进行业务处理,处理完成后不需要有返回值的情况下使用。
final Consumer<String> printString = System.out::println;
// 输出 "123!"
printString.accept("123!");
4.4.2 BiConsumer
源码:
@FunctionalInterface
public interface BiConsumer<T, U> {
/**
* Performs this operation on the given arguments.
*
* @param t the first input argument
* @param u the second input argument
*/
void accept(T t, U u);
default BiConsumer<T, U> andThen(BiConsumer<? super T, ? super U> after) {
Objects.requireNonNull(after);
return (l, r) -> {
accept(l, r);
after.accept(l, r);
};
}
}
使用场景: 接收 两个任意类型的参数 并且不返回任何结果的操作。通常是对传入的参数进行业务处理,处理完成后不需要有返回值的情况下使用。
// 传入一个字符串跟一个下标,函数内部将这两个参数拼起来输出。
final BiConsumer<String, Integer> printWithIndex = (str, index) -> System.out.println(index + ": " + str);
// 输出 "1: Hello"
printWithIndex.accept("Hello", 1);
4.4.3 DoubleConsumer
源码:
@FunctionalInterface
public interface DoubleConsumer {
/**
* Performs this operation on the given argument.
*
* @param value the input argument
*/
void accept(double value);
default DoubleConsumer andThen(DoubleConsumer after) {
Objects.requireNonNull(after);
return (double t) -> { accept(t); after.accept(t); };
}
}
使用场景: 接收 一个 double 类型的参数 并且不返回任何结果的操作。通常是对传入的参数进行业务处理,处理完成后不需要有返回值的情况下使用。
final DoubleConsumer print = System.out::println;
// 输出 "3.14"
print.accept(3.14);
4.4.4 IntConsumer
源码:
@FunctionalInterface
public interface IntConsumer {
/**
* Performs this operation on the given argument.
*
* @param value the input argument
*/
void accept(int value);
default IntConsumer andThen(IntConsumer after) {
Objects.requireNonNull(after);
return (int t) -> { accept(t); after.accept(t); };
}
}
使用场景: 接收 一个 int 类型的参数 并且不返回任何结果的操作。通常是对传入的参数进行业务处理,处理完成后不需要有返回值的情况下使用。
final IntConsumer printInt = System.out::println;
// 输出 "42"
printInt.accept(42);
4.4.5 LongConsumer
源码:
@FunctionalInterface
public interface LongConsumer {
/**
* Performs this operation on the given argument.
*
* @param value the input argument
*/
void accept(long value);
default LongConsumer andThen(LongConsumer after) {
Objects.requireNonNull(after);
return (long t) -> { accept(t); after.accept(t); };
}
}
使用场景: 接收 一个 long 类型的参数 并且不返回任何结果的操作。通常是对传入的参数进行业务处理,处理完成后不需要有返回值的情况下使用。
final LongConsumer printLong = System.out::println;
// 输出 "123"
printLong.accept(123L);
4.4.6 ObjDoubleConsumer
源码:
@FunctionalInterface
public interface ObjDoubleConsumer<T> {
/**
* Performs this operation on the given arguments.
*
* @param t the first input argument
* @param value the second input argument
*/
void accept(T t, double value);
}
使用场景: 接收 一个任意类型的参数 跟 一个 double 类型的参数 并且不返回任何结果的操作。通常是对传入的参数进行业务处理,处理完成后不需要有返回值的情况下使用。
final ObjDoubleConsumer<String> print = (str, val) -> System.out.println(str + ": " + val);
// 输出 "PI: 3.14"
print.accept("PI", 3.14);
4.4.7 ObjIntConsumer
源码:
@FunctionalInterface
public interface ObjIntConsumer<T> {
/**
* Performs this operation on the given arguments.
*
* @param t the first input argument
* @param value the second input argument
*/
void accept(T t, int value);
}
使用场景: 接收 一个任意类型的参数 跟 一个 int 类型的参数 并且不返回任何结果的操作。通常是对传入的参数进行业务处理,处理完成后不需要有返回值的情况下使用。
final ObjIntConsumer<String> print = (str, val) -> System.out.println(str + ": " + val);
// 输出 "Test: 42"
print.accept("Test", 42);
4.4.8 ObjLongConsumer
源码:
@FunctionalInterface
public interface ObjLongConsumer<T> {
/**
* Performs this operation on the given arguments.
*
* @param t the first input argument
* @param value the second input argument
*/
void accept(T t, long value);
}
使用场景: 接收 一个任意类型的参数 跟 一个 long 类型的参数 并且不返回任何结果的操作。通常是对传入的参数进行业务处理,处理完成后不需要有返回值的情况下使用。
final ObjLongConsumer<String> print = (str, val) -> System.out.println(str + ": " + val);
// 输出 "Timestamp: 123456789"
print.accept("Timestamp", 123456789L);